first thing you’d want to do is give the ball a name.
Let’s call it “ball”
Also, select the text where you want to display the score and give that a name as well.
Let’s call it “score”
In the default script, let’s create a few variables
window.points = 0;
score.setText(points);
what this does is it creates a window variable called “points” (which can be used anywhere in your code since it’s attached to the window), and sets the score text to the score in the beginning (which is zero).
Next up, let’s go to the prisoner, bill
. First thing I would want to point out is that when you click bill
he goes to the “hit” frame but never goes back to the normal one.
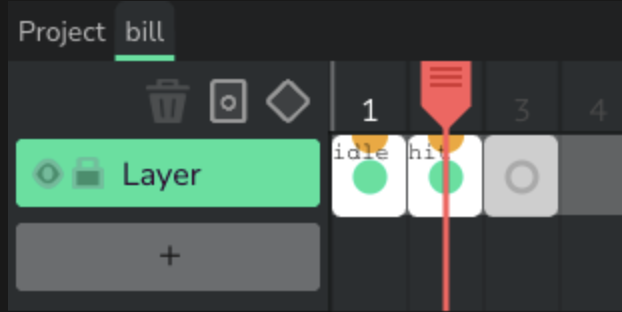
We want to go back to the “idle” once the hit animation is done. So first take a look at the hit animation… inside the clip of that frame
This animation goes up to 7 frames, and after those 7 the animation will loop back to frame 1.
Or at least it should…
I see you have set it to Play Once. Change it back to Loop
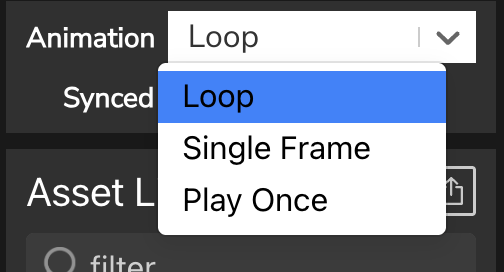
Rather than making it play once, we want it to loop so that the hitting animation plays again when we click on it. Now, let’s add an update script to this clip to check when the animation reaches frame 7, then set it back to frame 1 and go back to “idle.”
If you do this, then you should be able to click your prisoner as many times as you want and he’ll keep on trying to hit the ball.
Now, let’s go back to the ball.
Inside the ball clip, you have 3 different stages… “start”, “fail”, and “tang”
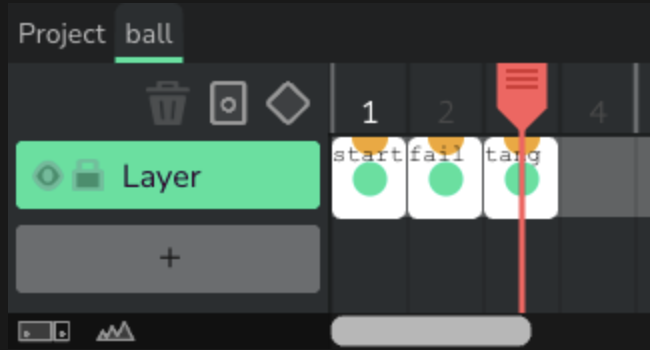
We know that when the prisoner, bill, is not in the “idle” frame and the ball is in start, we want to go to the “tang” animation inside the ball. However, there’s no way for us to tell when the “tang” animation ends.
So let’s go to the “tang” frame, and give the ball animation clip there a name. I’ll call it “ballAnim” (short for ball animation).
Do this for the “start” and “fail” animations as well. Go to each one and call the animation object there “ballAnim.”
Now let’s go back to the main project, and add an update script to the ball
clip.
The first thing you want to do is check if bill is currently on the “hit” frame and the ball is still on “start,” then have the ball go to the “tang” clip (hitting).
if(bill.currentFrameName === "hit" && this.currentFrameName == "start"){
this.gotoAndStop("tang");
}
Next, we want it so that when the hit animation is done, to check if bill is in “idle” (or not hitting) and to play the fail animation if that’s the case.
To check if the ball animation is done, you just need to see if we’re in the “tang” frame, and if the “ballAnim
” object (we named earlier) is on the first animation of the frame (which means it looped to the end and got back to the first frame). If the player, “bill”, is not trying to hit it at that point then we fail.
if(this.currentFrameName == "tang" && this.ballAnim.currentFrameNumber == 1){
if(bill.currentFrameName === "idle"){
this.gotoAndStop("fail");
}
}
Your code for the ball should look like this
Now the game should work. It might be a bit difficult since the ball animation is very quick, so I recommend making the ball animation longer.
Also, the “fail” animation in the ball loops. Go to that one and make it Play Once.
And lastly, you want the points to go up when you hit the ball.
Remember when we made the “window.points
” variable and I said this one is attached to the window, so we can use it anywhere in our code? That’s right.
Go inside the “tang” animation for the ball, and look at the second frame. Every time you hit the ball, the second frame will at least play. So every time that second frame plays, we know we hit the ball and therefore should get a “point.” So add a default script inside that frame, and increase the points
variable there.
(
points++
is a fancy way of saying “increase the points by 1”).
Your almost done— BUT we still don’t have the text object displaying the points.
Remember the text object, we named it “score” right? Go back to the Project timeline, and inside of an update script (could be inside bill
, ball
, or any clip in the main project really), write
score.setText(points);
This makes the “score” text object display the “points”.
And with that… the game is complete :)
I’ll send you a file in case you have difficulties walking through this tutorial.
prison.wick (164.9 KB)
I kinda coded this as I wrote this tutorial so it took a minute and I didn’t fully check what I wrote, but do let me know if anything I said sounds confusing. There should be around 7 hours left for your jam, I’ll be off for a moment but I’ll definitely be back so lemme know if you come by any trouble. Good luck!